Understand Recursion in a Simple Way
- Vish Katyan
- Jan 1, 2023
- 2 min read
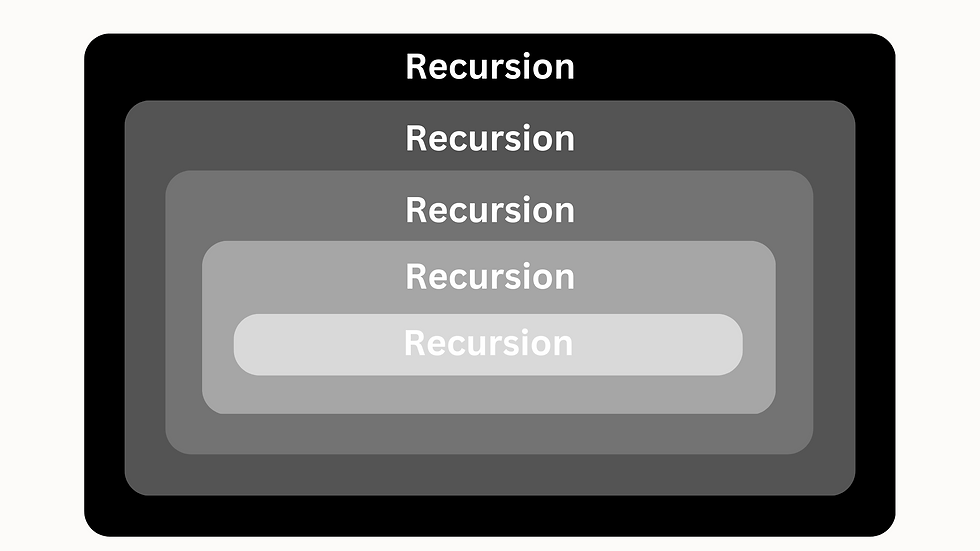
What is Recursion?
Instead of solving a problem iteratively, we can also solve it by recursion just to optimize the code. Iterative means to solve a problem through loops, sometimes there may be a problem that can solve by using a loop but it may cause confusion and the code is not as optimized as we want. Here we use recursion.
Now the question arises what is recursion? This is as simple as a function instead of not calling any other function to do any work, it calls itself.
A Function that calls itself is called Recursion. And the corresponding function is called Recursive Function.
Code that shows recursion:
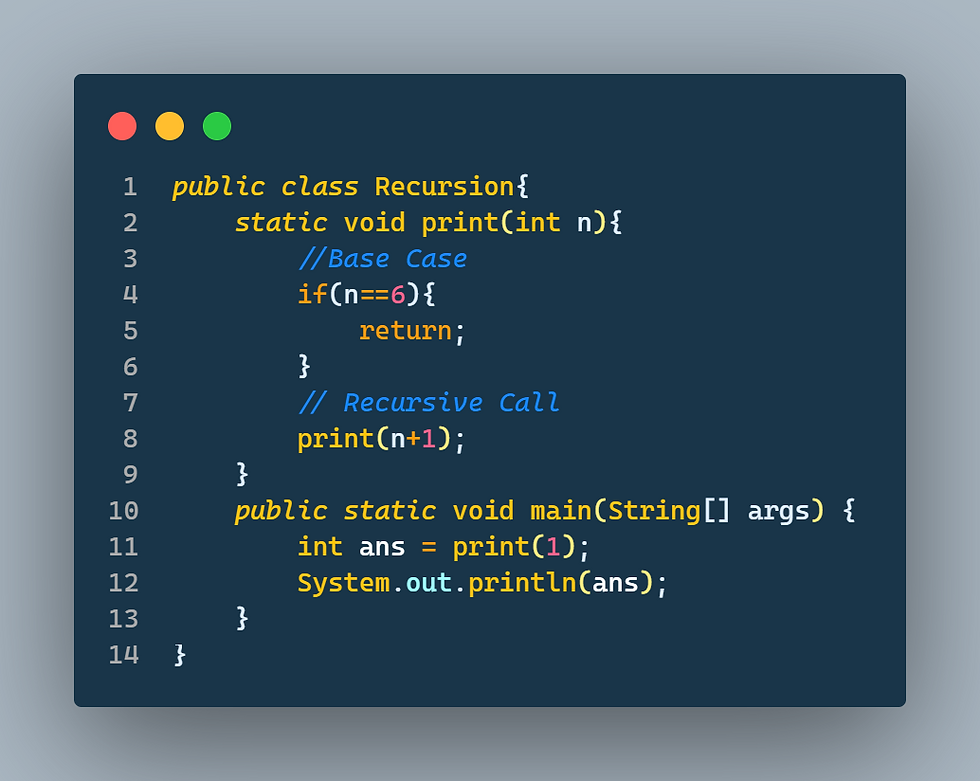
Why Recursion?
It helps in breaking down the bigger problem into small problems.
You can also convert recursive problems into iteration and vice-versa.
How are these functions stored in memory?
It uses more memory than iteration. At each recursive call, the function adds to the stack and holds its value until the call is finished. It uses a STACK data structure and stores in FILO or LIFO fashion.
Read More Blogs: How to Prepare for Array Data Structure for Placements?
What is Base Condition?
The base case is the condition in which you tell the function to stop its functionality and pop from the stack. It is also known as a terminating statement.
What if there is no base case?
The function will not be terminated and keep in a stack with new function calls so it leads to StackOverflow Error.
int fact(int n)
{
if (n < = 1) // base case
return 1;
else
return n*fact(n-1);
}
Hope that you have a basic idea about Recursion. Do check out the next blog to practice some easy-level problems.
Thank you : )
Kommentare